Problem
Alice in linear land
Statement
Alice lives on a line. Today, she will travel to some place in a mysterious vehicle. Initially, the distance between Alice and her destination is . When she input a number to the vehicle, it will travel in the direction of the destination by a distance of if this move would shorten the distance between the vehicle and the destination, and it will stay at its position otherwise. Note that the vehicle may go past the destination when the distance between the vehicle and the destination is less than .
Alice made a list of numbers. The number in this list is . She will insert these numbers to the vehicle one by one.
However, a mischievous witch appeared. She is thinking of rewriting one number in the list so that Alice will not reach the destination after moves.
She has plans to do this, as follows:
Rewrite only the number in the list with some integer so that Alice will not reach the destination.
Write a program to determine whether each plan is feasible.
Constraints
and each are integers.
Input
Input is given from Standard Input in the following format:
Output
Print lines. The line should contain YES
if the plan is feasible, and NO
otherwise.
Sample
Input #1
1 | 4 10 |
Output #1
1 | NO |
Explanation #1
For the first plan, Alice will already arrive at the destination by the first three moves, and therefore the answer is NO
. For the second plan, rewriting the third number in the list with will prevent Alice from reaching the destination as shown in the following figure, and thus the answer is YES
.
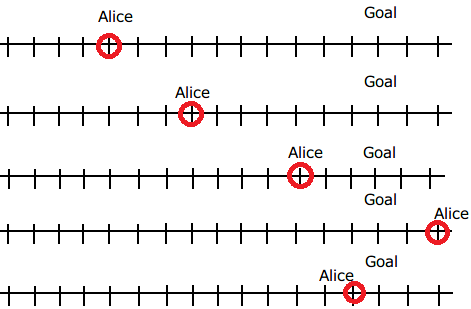
Input #2
1 | 5 9 |
Output #2
1 | YES |
Explanation #2
Alice will not reach the destination as it is, and therefore all the plans are feasible.
Input #3
1 | 6 15 |
Output #3
1 | NO |
标签:DP
Translation
有一个无限长的数轴,你现在在处,有条指令,每条指令为一个整数。顺次处理每条指令,如果你当前在处,你会走到处。有条询问,对于每条询问,回答能否通过改变的值使得最后不能到达。
Solution
精妙的做法,有点类似构造,又有点像。
首先处理出数组,其中表示在没有更改指令的情况下,处理前条指令后的位置。那么如果能够修改,我们可以在处理完后到达中的所有位置。我们需要找到一个数组,其中表示修改并处理完后,至少要在哪个位置才能使处理完后面的指令后不能到达。显然,如果,一定能找到可行方案,否则一定不能。
考虑如何得到。我们倒序计算。首先,显然有。不难发现一定是递减的,于是倒序计算时我们用前面的值作为基础值来处理出现在处理到的。对于,不小于,如果,那么从位置进行指令一定不会走到更小的点上,因此可以取到其最小值;否则,需要使其操作后不小于,因此。
处理出后判断即可。时间复杂度。
Code
1 |
|